button
A customizable button component for triggering actions in your application.
Features
- Customizable: Easily adjust the button's variant, size, and appearance.
- Keyboard Shortcuts: Assign keyboard shortcuts for enhanced user interactions.
- Tooltip Integration: Display tooltips with custom delay and content.
- Icon Support: Include primary and secondary icons alongside text or as standalone elements.
- Collapsible: Optionally collapse the button to icon-only mode.
- Loading State: Display a loading spinner while actions are in progress.
- Label Support: Add labels with flexible positioning and style.
Installation
npx duck-ui@latest add button
npx duck-ui@latest add button
Usage
import { Button } from '@/components/ui/button'
import { Button } from '@/components/ui/button'
<Button>Button</Button>
<Button>Button</Button>
Button
Button
is a versatile and customizable React component designed to render a button with various styles and functionalities. It supports different sizes, variants, and additional features like loading states and icons. The component is flexible and can be used in a variety of scenarios, from simple buttons to complex interactive elements.
Example Usage:
import { Button } from '@/components/ui/button'
const MyButton = () => {
return (
<Button
size="large"
variant="primary"
label={{
children: 'Submit',
side: 'left',
showLabel: true,
}}
icon={<Icon />}
onClick={() => console.log('Button clicked')}
>
Submit
</Button>
)
}
import { Button } from '@/components/ui/button'
const MyButton = () => {
return (
<Button
size="large"
variant="primary"
label={{
children: 'Submit',
side: 'left',
showLabel: true,
}}
icon={<Icon />}
onClick={() => console.log('Button clicked')}
>
Submit
</Button>
)
}
Link
You can use the buttonVariants
helper to create a any element like a link that looks like a button.
import { buttonVariants } from '@/components/ui'
import { buttonVariants } from '@/components/ui'
<Link className={buttonVariants({ variant: 'outline' })}>I'am a Link 🦆</Link>
<Link className={buttonVariants({ variant: 'outline' })}>I'am a Link 🦆</Link>
Alternatively, you can set the asChild
parameter and nest the link component.
<Button asChild>
<Link href="/tos">I'am a Link with tos 🦆</Link>
</Button>
<Button asChild>
<Link href="/tos">I'am a Link with tos 🦆</Link>
</Button>
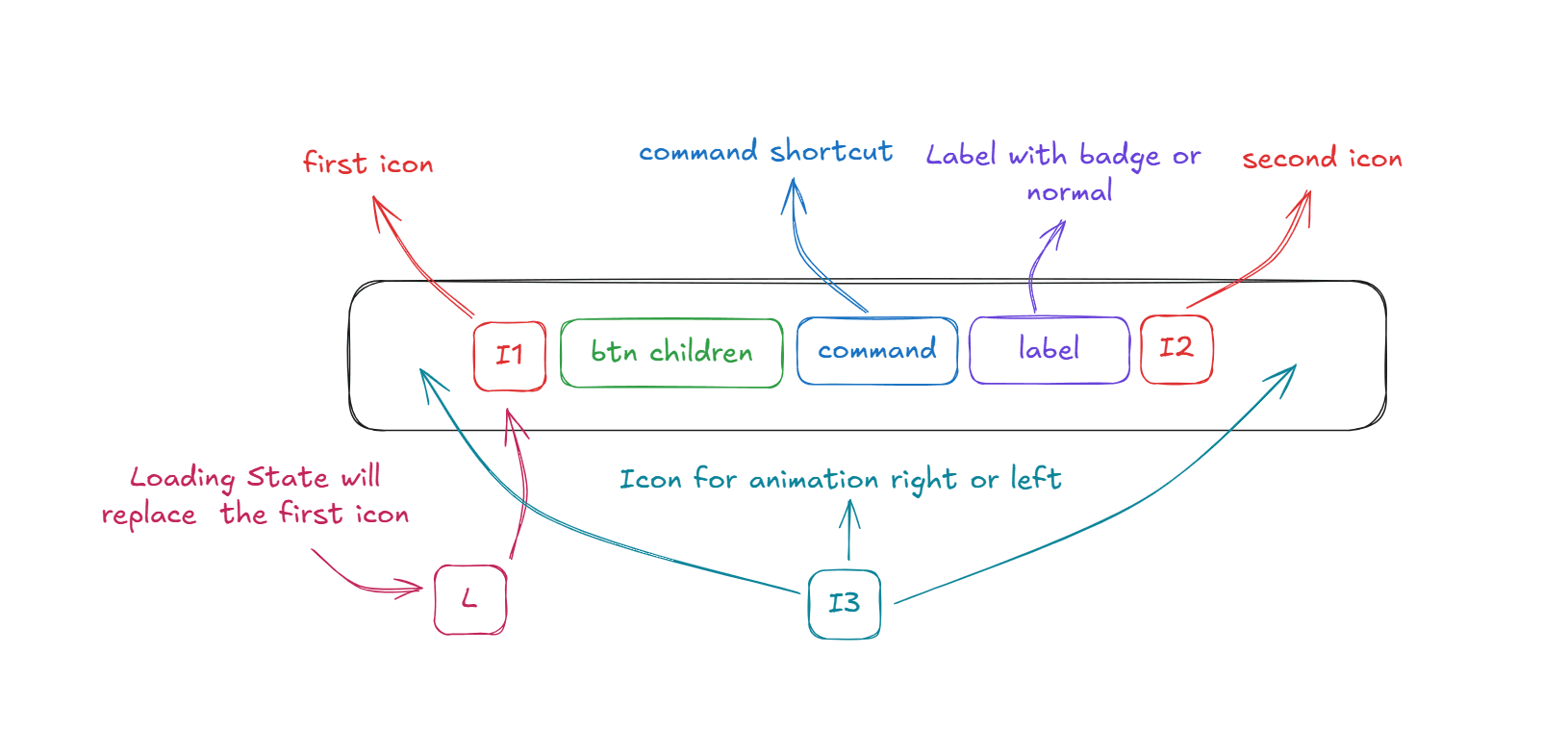
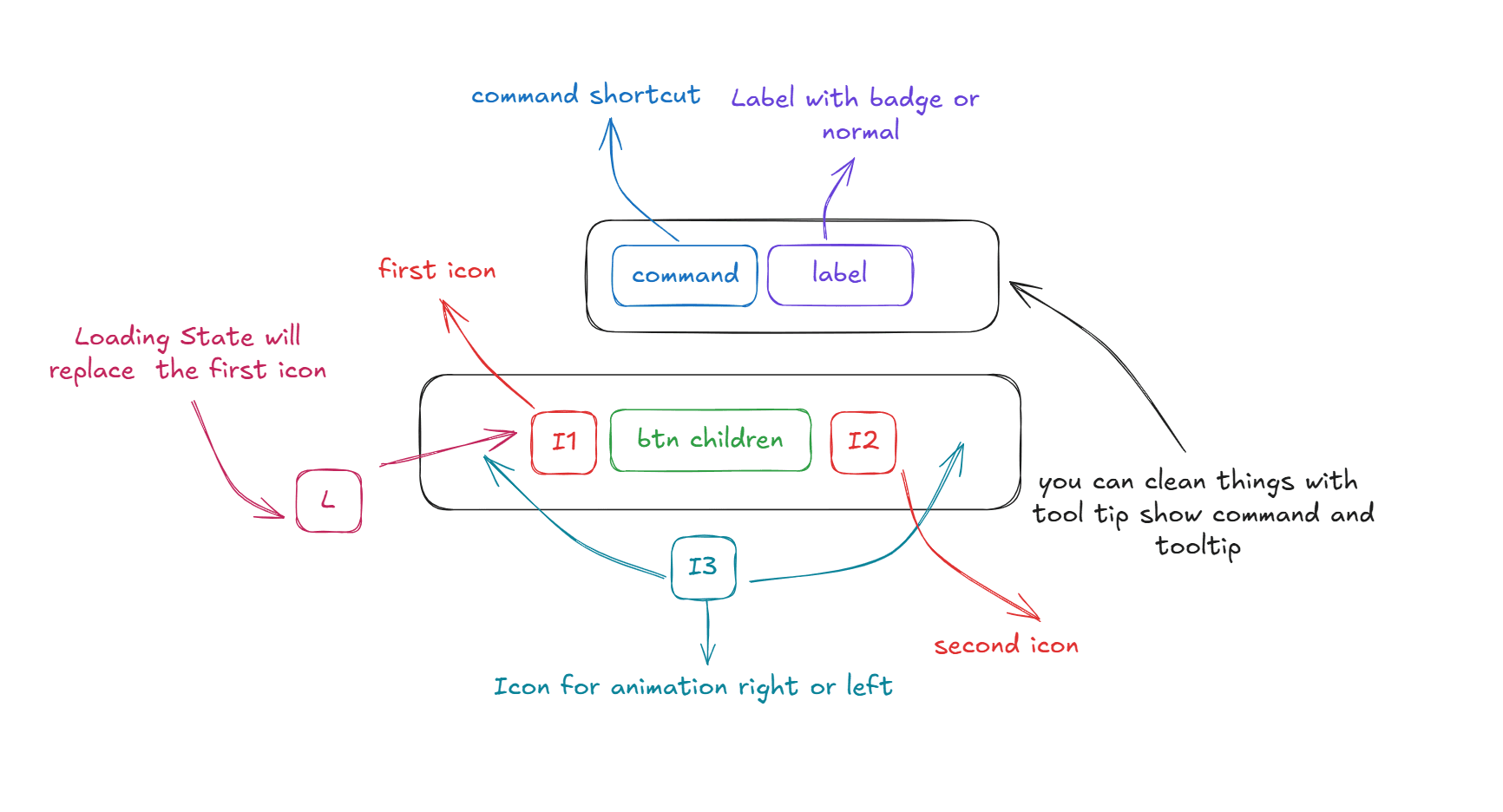
Examples
Primary
Secondary
Ghost
Outline
Link
Destructive
Warning
Dashed
Nothing
With Border
With Border Destructive
With Border Warning
With Border Secondary
With Icon
Loading
With Second Icon
With Badge Label
With Label
With tooltip
with Command
Command Tooltip
Collapsible
Expand Icon Right
Expand Icon Left
Ring Hover
Gooey Right
Gooey Left
Link Hover 1
Link Hover 2
Advanced
API Reference
General Button Properties
-
asChild
(boolean
, optional):- Renders the button as a child element of another component when set to
true
, allowing the button to inherit styling from the parent component.
- Renders the button as a child element of another component when set to
-
isCollapsed
(boolean
, optional):- Collapses the button into an icon-only state, typically used for compact views or toggling states.
-
size
('default' | 'xs' | 'sm' | 'sm' | 'lg' | 'xl' | 'xxl' | 'xxxl' | 'icon'
, optional):- Sets the button size. Defaults to
'default'
. Other options:'xs'
: ExtraSmall button with reduced padding.'sm'
: Small button with reduced padding.'default'
: Defulat button size with reduced padding.'lg'
: Large button with extra padding.'xl'
: XLarge button with extra padding.'xxxl'
: XXLarge button with extra padding.'xxxl'
: XXXLarge button with extra padding.'icon'
: Icon-only button without additional padding.
- Sets the button size. Defaults to
-
border
('default' | 'primary' | 'secondary' | 'destructive' | 'warning'
, optional):- Sets the button size. Defaults to
'default'
. Other options:'default'
: Without a border.'secondary'
: With a secondary border for optional actions.'destructive'
: With a destructive border for danger.'warning'
: With a warning border for warning.
- Sets the button size. Defaults to
-
variant
(string
, optional):- Defines the visual style of the button. Available options:
default
: Primary button style.destructive
: Red, indicating a destructive action.warning
: Yellow, indicating a warning action.secondary
: secondary button with no fill.outline
: Outlined button with no fill.dashed
: Dashed button with no fill.ghost
: Transparent with hover effects.link
: Styled as a link.expand_icon
: For expandable buttons.- Special effect variants:
ring_hover
,shine
,gooey_right
,gooey_left
,link_hover1
,link_hover2
- Defines the visual style of the button. Available options:
-
border
(string
, optional):- Defines the visual style of the button border. Available options:
default
: No border style.primary
: Primary border style.destructive
: Destructive border style.warning
: Warning border style.secondary
: secondary border style.
- Defines the visual style of the button border. Available options:
-
loading
(boolean
, optional):- When
true
, displays a loading spinner (usesLoader
from Lucide).
- When
-
children
(React.ReactNode
):- Content or text inside the button.
-
...props
(React.ReactNode
):- the rest of props on an HTML button.
Icon Properties
Button icons can be configured for single or dual icon use, and include optional animation.
Primary Icon
icon
(IconType
, optional):- Specifies the primary icon displayed within the button.
Secondary Icon
secondIcon
(IconType
, optional):- Adds a secondary icon to appear alongside the primary icon.
Animation Icon
animationIcon
({ icon: [IconType](#icontype), iconPlacement: 'left' | 'right' }
, optional):- Defines an animated icon that appears on hover, with
iconPlacement
specifying its position.
- Defines an animated icon that appears on hover, with
Type Definitions
IconType
Defines an icon component from the Lucide library.
children
(LucideIcon): The icon component.- Additional SVG Props: Includes all
React.SVGProps
for SVG customization.
LabelType
Configures an optional label with tooltip support for additional context.
showCommand
(boolean
, optional): Show the command on theTooltip
.showLabel
(boolean
, optional): Show the label on theTooltip
.delayDuration
(number
, optional): The delay theTooltip
gonna take to me mounted....BadgeProps
(BadgeType
,optional): The rest ofBadge
component props....TooltipProps
(TooltipType
,optional): The rest ofTooltip
component props....TooltipContent
(TooltipContentType
,optional): The rest ofTooltipContent
component props.
CommandType
Provides keyboard command handling and displays an associated shortcut or action label, it defines a command for keyboard shortcuts, with an action function, state management, and label.
label
(string
, optional): The label for theCommand
.key
(string
, optional): The key for theCommand
.show
(boolean
, optional): To show theCommand
body on the button.action
(() => void
): The function that executes with theCommand
.shortcut
(string[]
, optional): The keyboard keys associated with theCommand
....BadgeProps
(optional): The rest ofBadge
components props.
Changelog
2024-12-19
- New Variants: Added new button variants, including muted, dashed, and warning styles.
- Border Props: Introduced new button border properties to allow borders with hover effects.
- Hook Update: Removed state from the
useDuckShortcut
hook, previously imported from@ahmedayoub/shortcut
. - Documentation: Updated documentation to reflect the latest changes to the Button component.